First we need a platform that the user control. Our platform will be a BarCallbacks with key handler. To control the movement of the objects we will introduce a new object 'World' which will handle the movement code. The world object is a timer object that will tick every few seconds and recalculate the coordinates of the registered vectors and redisplay the screen. Also the VectorBox object now will have distance and angle making it a real vector. VectorBox object here :
1: @interface VectorBox : NSObject {
2: float x, y, xr, yr, distance, angle;
3: }
4: @property float x, y, xr, yr, distance, angle;
5: -(id) initWithX:(float)_x y:(float)_y xr:(float)_xr yr:(float)_yr;
6: -(id) initWithX:(float)_x y:(float)_y xr:(float)_xr yr:(float)_yr distance:(float) _d angle:(float) _a;
7: @end
Distance will determine how many pixels will the object travel with the tick of the clock. Angle is at what angle will the object move. The init function without the distance and angle will set these values to zero. And the World object :
1: @interface World : NSObject {
2: NSMutableArray * boxes;
3: NSUInteger milis;
4: }
5: @property (readonly) NSUInteger milis;
6: @property (readonly) NSMutableArray * boxes;
7: -(id) addVectorBox:(VectorBox *) vbox;
8: -(id) startTickingEveryMilis:(NSUInteger) milis;
9: +(id) world;
10: void worldTick(int value);
11: @end
The 'boxes' parameter will hold the vectors that will be animated. The 'milis' parameter is the number of milliseconds that our code will wait before firing to animate our vectors. addVectorBox method adds new vectors and startTickingEveryMilis method sets the timer. The world object is to be used as a 'singleton', one copy of it should be available at any time, and to access that instance we will use the static 'world' method. worldTick function is the our glut timer callback function. Its registered first as the startTickingEveryMilis method is called. worldTick function :1: void worldTick(int value) {
2: World * world = [World world];
3: int len = [world.boxes count];
4: for( int i = 0; i < len; i++) {
5: VectorBox * vbox =[world.boxes objectAtIndex:i];
6: vbox.x += vbox.distance*cos(vbox.angle);
7: vbox.y += vbox.distance*sin(vbox.angle);
8: }
9: glutPostRedisplay();
10: glutTimerFunc(world.milis,worldTick,1);
11: }
It traverses of the boxes array and moves them using a simple trigonometric function and updates the coordinate values. Then the glutPostRedisplay function is called so the screen is redrawn. Using the glutTimerFunc we reregister our callback function. PlatformCallbacks extends from the BarCallbacks it just adds the key handler code to it.1: -(void) keyHandler:(unsigned char) key x:(int) x y:(int) y {
2: if(key =='d') {
3: box.angle = 0;
4: box.distance = distance;
5: } else if(key =='a') {
6: box.angle = M_PI;
7: box.distance = distance;
8: }
9: }
10: -(void) keyUp:(unsigned char) key x:(int) x y:(int) y {
11: box.distance = 0;
12: }
Glut calls the keyHandler function as long as the key is pressed. So when the 'd' key is pressed we will set the registered vectors distance to move right. keyUp function is called when the key is released so we will use it to set the vectors distance to zero thus making it stop.Now we need to implement our bouncing ball. First thing I am going to do is refactoring the code so that we may have a common base class for BarCallbacks and BallCallbacks. I will call it the BaseCallbacks. All my callbacks will extend from this base. OpenGL does not have circle driving function by default so we need to come up with a approximate formula :
@implementation BallCallbacks
-(void) display {
// loads the identity matrix
glLoadIdentity();
glColor3f(1.0,0.0,0.0);
glBegin(GL_POLYGON);
for (int i = 0; i < 360; i++) {
float x1 = (cos((M_PI*i)/180) * box.xr) + box.x;
float y1 = (sin((M_PI*i)/180) * box.yr) + box.y;
glVertex3f(x1,y1,0);
}
glEnd();
}
@end
Using glBegin function we start drawing vertex to vertex. Our circle is actually a polygon with 360 vertexes. We end drawing with glEnd function. After initializing with distance and angle our ball will start moving. As its now our ball will pass through our walls. To make it bounce we need to detect collision and change the angle appropriately. We will be checking for collisions at every tick of the clock in the World object : void worldTick(int value) {
World * world = [World world];
int len = [world.boxes count];
for( int i = 0; i < len; i++) {
VectorBox * vbox =[world.boxes objectAtIndex:i];
vbox.x += vbox.distance*cos(vbox.angle);
vbox.y += vbox.distance*sin(vbox.angle);
}
for( int i = 0; i < len; i++) {
VectorBox * vbox =[world.boxes objectAtIndex:i];
if( !vbox.changesAngleAfterCollision) {
continue;
}
for( int j = 0; j < len; j++) {
if( i == j) {
continue;
}
VectorBox * obox =[world.boxes objectAtIndex:j];
[vbox detectCollision:obox];
}
}
glutPostRedisplay();
glutTimerFunc(world.milis,worldTick,1);
}
We check for collision with objects which changes its angle after collision. In our case we only need to check collision of ball and the other objects. -(id) detectCollision:(VectorBox *) obox {
// check if this object collides with other from bottom
if(obox.x + obox.xr > x && obox.x - obox.xr < x ) {
if(obox.y + obox.yr > y + yr && obox.y - obox.yr < y + yr) {
angle = 2*M_PI - angle;
}
}
if(obox.x + obox.xr > x && obox.x - obox.xr < x ) {
if(obox.y + obox.yr > y - yr && obox.y - obox.yr < y - yr) {
angle = 2*M_PI - angle;
}
}
if(obox.y + obox.yr > y && obox.y - obox.yr < y ) {
if(obox.x + obox.xr > x + xr && obox.x - obox.xr < x + xr) {
angle = 3*M_PI - angle;
}d
}
if(obox.y + obox.yr > y && obox.y - obox.yr < y ) {
if(obox.x + obox.xr > x - xr && obox.x - obox.xr < x - xr) {
angle = 3*M_PI - angle;
}
}
return self;
}
This works pretty smoothly most of the time but sometime ball gets stuck.This is how it looks like in the end :
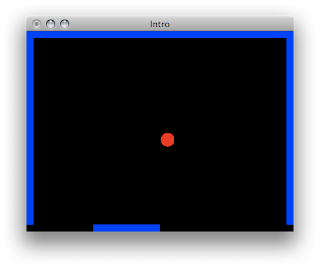
No comments:
Post a Comment